Key Cave Adventure Game Using Python
- Codersarts AI
- Sep 25, 2020
- 5 min read
Gameplay
Key Cave Adventure Game is a single-player dungeon-crawler game where the player adventurously explores a dungeon. The objective is for the player to find the key and get out of the dungeon through the door. This game is full of adventure. The game play utilises simple key commands. The positions of Entities are always represented as (row, col). The game’s grid should always be a square.
Commands
Table 1. List of valid actions.
Input Action
‘-DIRECTION-’ Direction to move in
‘I -DIRECTION-’ ‘I W’ would investigate the Entity in the given direction. ‘H’ Help text (see examples at end)
‘Q’ Quit
Table 2. List of valid Directions.
Input Direction
‘W’ Up
‘S’ Down
‘A’ Left
‘D’ Right
Table 3. List of characters used in the game
Character Description
‘#’ WALL
‘O’ PLAYER
'K' KEY
'D' DOOR
‘ ’ SPACE
The Player can move within the dungeon by using any of the valid direction inputs outlined in Table 2. The Player must collect the KEY by walking into it and then the DOOR can be unlocked.
Game over
The Player wins the game by collecting the KEY and heading to the DOOR. This message should display: You have won the game with your strength and honour!
If the Player runs out of moves before they collect the KEY and unlock the DOOR then the Player loses the game. This message should display: You have lost all your strength and honour.
The player loses a move count when they:
Successfully moving to a new position.
Unsuccessfully moving to a new position. E.g. The new position is a Wall.
Investigating an Entity in a given direction
As seen in the Example game play.
Getting Started
Files
a2.py - file where you write your submission
gamen.txt - the nth dungeon layout. There will be multiple provided. “game1.txt” is the best one to start from
To start, download a2_files.zip from Blackboard and extract the contents. This folder contains all the necessary files to start this assignment. You will be required to implement your assignment in a2.py. Do not modify any files from a2.zip except a2.py. The only file that you are required to submit is a2.py. Some support code has been provided to assist with implementing the tasks
Classes
Many modern software programs are implemented with classes and this assignment will give you some practice in working with them. The class structure that you will be working with is shown diagrammatically in Figure 1.
In Figure 1 an arrow with a solid head indicates that one class stores an instance of another class. (This is analogous, say, to a list storing instances of strings within it, say, sample_list=[‘dog’, ‘cat’]). In Figure 1, for example, GameApp points to Display meaning that GameApp contains an instance of Display. An arrow with an open head indicates that one class is a subclass of another class. I.e. one class inherits from another. E.g. Item is a subclass of Entity.
You can add classes to reduce code duplication. This can include adding your own private helper methods. However, you must include the classes outlined below
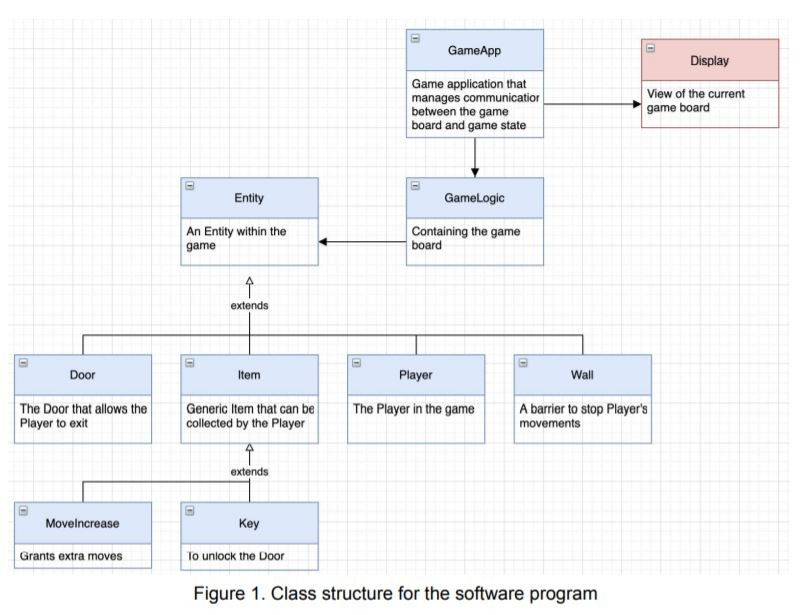
Entity
A generic Entity within the game. Each Entity has an id, and can either be collided with (two entities can be in the same position) or not (two entities cannot be in the same position.) The collidable attribute should be set to True for an Entity upon creation. Entity should be constructed with Entity(). Entity should have the following methods defined:
get_id(self) -> str: Returns a string that represents the Entity’s ID.
set_collide(self, collidable: bool): Set the collision state for the Entity to be True.
can_collide(self) -> bool: Returns True if the Entity can be collided with (another Entity can share the position that this one is in) and False otherwise.
__str__(self) -> str: Returns the string representation of the Entity. See example output below.
__repr__(self) -> str: Same as str(self).
Examples
>>> entity = Entity()
>>> entity.get_id()
'Entity'
>>> entity.can_collide()
True
>>> str(entity)
"Entity('Entity')"
>>> repr(entity)
"Entity('Entity')"
>>> entity = Entity()
>>> entity.can_collide()
True
>>> entity.set_collide(False)
>>> entity.can_collide()
False
(Note that Entity should never be set to False, this is just an example)
Wall
A Wall is a special type of an Entity within the game. The Wall Entity cannot be collided with. Wall should be constructed with Wall(). Wall should have the following methods defined:
get_id(self) -> str: Returns a string that represents the Wall’s ID.
set_collide(self, collidable: bool): Set the collision state for the Wall to be False.
can_collide(self) -> bool: Returns True if the Wall can be collided with and False otherwise.
__str__(self) -> str: Returns the string representation of the Wall. See example output below.
__repr__(self) -> str: Same as str(self).
Examples
>>> wall = Wall()
>>> wall.get_id()
'#'
>>> wall.can_collide()
False
>>> str(wall)
"Wall('#')"
>>> repr(wall)
"Wall('#')"
>>> wall.set_collide(True)
>>> wall.can_collide()
True
(Note that Wall should never be set to True, this is just an example)
Item
An Item is a special type of an Entity within the game. This is an abstract class. By default the Item Entity can be collided with. Item should be constructed with Item(). Item should have the following methods defined:
get_id(self) -> str: Returns a string that represents the Item’s ID.
set_collide(self, collidable: bool): Set the collision state for the Item to be True.
can_collide(self) -> bool: Returns True if the Item can be collided with and False otherwise.
on_hit(self, game: GameLogic): This function should raise the NotImplementedError
Examples
>>> game = GameLogic()
>>> item = Item()
>>> item.can_collide()
True
>>> item.on_hit(game)
Traceback (most recent call last):
File "<pyshell#30>", line 1, in <module>
item.on_hit(game)
File "/Users/.../a2.py", line 75, in on_hit
class Item(Entity):
NotImplementedError
>>> str(item)
"Item('Entity')"
>>> repr(item)
"Item('Entity')"
Key
A Key is a special type of Item within the game. The Key Item can be collided with. Key should be constructed with Key(). Key should have the following methods defined:
get_id(self) -> str: Returns a string that represents the Key’s ID.
set_collide(self, collidable: bool): Set the collision state for the Key to be True.
can_collide(self) -> bool: Returns True if the Key can be collided with and False otherwise.
on_hit(self, game: GameLogic) -> None: When the player takes the Key the Key should be added to the Player’s inventory. The Key should then be removed from the dungeon once it’s in the Player’s inventory.
(hint: Move onto the Player and GameLogic class and come back to this when they are partly implemented.)
__str__(self) -> str: Returns the string representation of the Key. See example output below.
__repr__(self) -> str: Same as str(self)
Examples
>>> key = Key()
>>> key.get_id()
'K'
>>> key.can_collide()
True
>>> str(key)
"Key('K')"
>>> repr(key)
"Key('K')"
(below are example of the on_hit() method)
>>> game = GameLogic()
>>> game.get_game_information()
{(1, 3): Key('K'), (3, 2): Door('D'), (0, 0): Wall('#'), (0, 1):
Wall('#'), (0, 2): Wall('#'), (0, 3): Wall('#'), (0, 4): Wall('#'),
(1, 0): Wall('#'), (1, 2): Wall('#'), (1, 4): Wall('#'), (2, 0):
Wall('#'), (2, 4): Wall('#'), (3, 0): Wall('#'), (3, 4): Wall('#'),
(4, 0): Wall('#'), (4, 1): Wall('#'), (4, 2): Wall('#'), (4, 3):
Wall('#'), (4, 4): Wall('#')}
>>> player = game.get_player()
>>> player.get_inventory()
[]
>>> player.set_position((1,3))
>>> key.on_hit(game)
>>> player.get_inventory()
[Key('K')]
>>> game.get_game_information()
{(3, 2): Door('D'), (0, 0): Wall('#'), (0, 1): Wall('#'), (0, 2):
Wall('#'), (0, 3): Wall('#'), (0, 4): Wall('#'), (1, 0): Wall('#'),
(1, 2): Wall('#'), (1, 4): Wall('#'), (2, 0): Wall('#'), (2, 4):
Wall('#'), (3, 0): Wall('#'), (3, 4): Wall('#'), (4, 0): Wall('#'),
(4, 1): Wall('#'), (4, 2): Wall('#'), (4, 3): Wall('#'), (4, 4):Wall('#')}
Output:
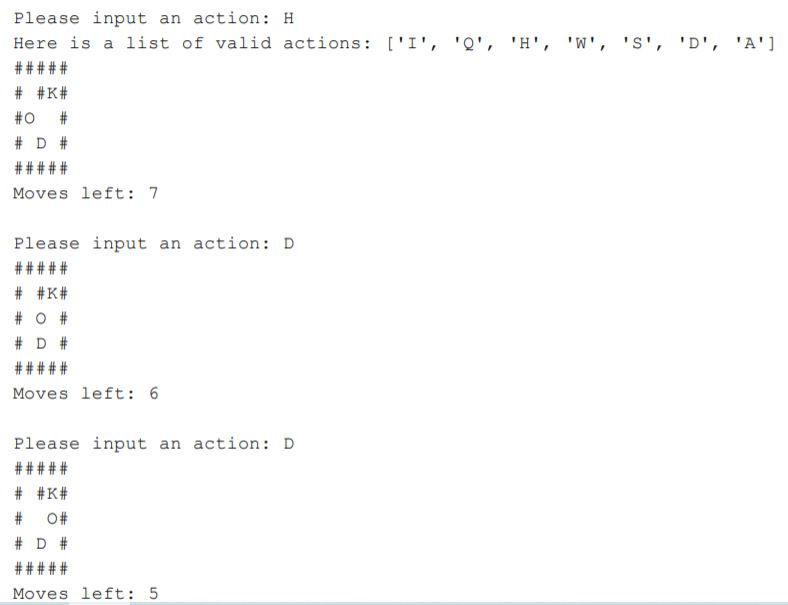
If you need complete solution of this game then send your details at below id:
contact@codersarts.com
Comments