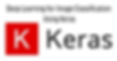
In this article we will discuss Convolutional neural networks and perform image classification using keras.
What is CNN ?
CNN stands for convolutional neural networks. Convolutional is one type of scanning and the Neural network is a system of interconnected artificial neurons that exchange messages to each other. Nowadays CNN is widely used for pattern and image recognition techniques. They have a number of advantages compared to other techniques. Hence so many companies use these techniques.
How does it work ?
Machines identify the image as a pixel form and do these pixel scanning by filter and collect information of the input image. When we slide the filter on the image we get new values by matching from the pixel value. These values help us to identify all the image features. Image features are fed into the convolutional layer. Outputs of convolutional layers are fed into a fully connected layer. Finally CNN predicts the features of the image and we get the result.
Different layers of CNN
Convolutional layer
Poling layer
Fully connected layer
Why is CNN required ?
It gives high accuracy
It require less memory for processing and execution
Image detection using CNN is robust to distortion like shape change due to camera lens, different position, different lighting conditions etc.
Now we are going to perform the Chest X-Ray image classification using Keras.

We have a dataset of Chest X-Ray images (pneumonia). We will perform the image classification using keras. Keras is a deep learning API written in Python, running on top of the machine learning platform TensorFlow. It is an open-source software library that provides a Python interface for artificial neural networks. It is an open-source software library that provides a Python interface for artificial neural networks. Keras follows best practices for reducing cognitive load: it offers consistent & simple APIs, it minimises the number of user actions required for common use cases, and it provides clear & actionable error messages. It also has extensive documentation and developer guides.
First we will import some important libraries. We have a dataset of chest X-Ray images which is downloaded from kaggle. The Train dataset contains a total of 5216 images, where 1341 images belong to the Normal category, 3875 images depicted Pneumonia And, Test dataset contains a total of 624 images, where 234 images belong to the normal category, 390 images depicted Pneumonia.
# Importing all necessary libraries
from keras.preprocessing.image import ImageDataGenerator
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D
from keras.layers import Activation, Dropout, Flatten, Dense
from keras import backend as K
import matplotlib.pyplot as plt
from os
from os.path
from pathlib import *
import pandas as pd
import seaborn as sns
Create neural network models and to train a dataset. The architecture of a CNN is a key factor in determining its performance and efficiency. The way in which the layers are structured, which elements are used in each layer and how they are designed will often affect the speed and accuracy with which it can perform various tasks.
The function Sequential( ) is used to group a linear stack of layers into a tf.keras.Model. We defined an instance of Sequential named 'model' and using it we added 3 hidden layers to the neural network. The first two layers have 'relu' as their activation function and the last one has 'softmax' as its activation function. We then combined all the layers using model. compile and trained the model on our training data.
Convolutional layer
Apply Convolutional operation on the input data using conv_2d library and passing to the MaxPooling 2 layer. Conv_2d converts the image into multiple images. Activation is the activate function. This layer computes the dot product between their weights and a small region and they are connected to the input layer. It will change the dimension depending on the filter size.
Relu
Relu is a Rectifying Linear Unit. The Relu function returns the positive value or 0 in place of the previous negative value. It does not change the dimension of the previous layer.
Max Pooling layer
Apply max_pool_2d on the convolutional layer. This layer will reduce the spatial size of the convolved features and also reduce overfitting by providing an abstract representation of them. Below is an example of a max pooling layer.

Fully connected layer
This layer is the final layer of the CNN model. This layer computes all the input layers and determines the final prediction. In this layer all the input layer nodes are connected to every node in the second layer. We can use one or more than fully connected layers.
Dropout layer
Dropout layer is a regularization technique and it is used for reducing overfitting in CNN. In general the dropout layer is used in fully connected layers in deep learning models but it is possible to use after the max_pooling layer. Dropout layer should not be used on test data because dropout is a random process of disabling the neuron in a layer and your network will have different output every sequence of activation.
Code Snippet :
model = Sequential()
model.add(Conv2D(32, (2, 2), input_shape=input_shape))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(32, (2, 2)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(64, (2, 2)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(32, (2, 2)))
model.add(Activation('relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(64))
model.add(Activation('relu'))
model.add(Dropout(0.5))
model.add(Dense(1))
model.add(Activation('sigmoid'))
model.compile(loss='binary_crossentropy',optimizer='rmsprop',metrics=['accuracy'])
model.summary()
Once a model is "built", you can call its summary() method to display its contents However, it can be very useful when building a Sequential model incrementally to be able to display the summary of the model so far, including the current output shape. In this case, you should start your model by passing an Input object to your model, so that it knows its input shape from the start

We will plot a graph of the number of pneumonia affected X-Ray images and normal images present in the training dataset.
Code Snippet :
# count plot of training dataset
sns.set_style('whitegrid')
sns.countplot(x='Label',data=train_df)
In the following count plot we can see the dataset contains pneumonia affected X-Ray are over 3500 and Normal X-Rays are below 1500.
Output :

We will plot some images which is present in the dataset
Code snippet :
# Display some pictures of the dataset
fig, axes = plt.subplots(nrows=4, ncols=6, figsize=(15, 7),subplot_kw={'xticks': [], 'yticks': []})
for i, ax in enumerate(axes.flat):
ax.imshow(plt.imread(train_df.Filepath[i]))
ax.set_title(train_df.Label[i], fontsize = 15)
plt.tight_layout(pad=0.5)
plt.show()
Output :

We will now check the accuracy and loss of the model and will plot the same.
Code Snippet :
# Plot graph
plt.plot(history.history['accuracy'],'r',label='training accuracy')
plt.plot(history.history['val_accuracy'],'b',label='validation accuracy')
plt.xlabel('epochs')
plt.ylabel('accuracy')
plt.legend()
plt.show()
plt.plot(history.history['loss'],'r',label='training loss')
plt.plot(history.history['val_loss'],'b',label='validation loss')
plt.xlabel('epochs')
plt.ylabel('loss')
plt.legend()
plt.show()
Output :

Thank You