Python Programming Help - Python Tkinter Tutorial
- Codersarts AI
- Aug 8, 2019
- 5 min read
Tkinter Tutorial

Hi Everyone!
Welcome to my Course Python Tkinter Tutorial
In this course, we are going to learn how to do GUI in python.
I am a python developer for a long time, and I’m so excited to share my knowledge of this awesome programming Language.
This course is designed for both beginner and professional, and also helpful for everyone who wish to learn the GUI Programming in Python Tkinter.
I hope you’ll join me on this journey to learn Python with the python: Getting started courses at CodersArts.
In this series of python learning. We will discuss the following topics
What is a Graphical User Interface (GUI) in Python?
Libraries use in Python Tkinter
What is Tkinter?
Fundamentals of Tkinter Using Python
Tkinter Widgets in Python
Geometry Management in Python
Organization of Layouts and Widgets in Python Tkinter
Binding Functions in Python
What is a Graphical User Interface (GUI) in Python?
Stands for "Graphical User Interface" and is pronounced "gooey." It is a user interface that includes graphical elements, such as windows, icons and buttons. The term was created in the 1970s to distinguish graphical interfaces from text-based ones, such as command line interfaces. However, today nearly all digital interfaces are GUIs.
The first commercially available GUI, called "PARC," was developed by Xerox. It was used by the Xerox 8010 Information System, which was released in 1981. After Steve Jobs saw the interface during a tour at Xerox, he had his team at Apple develop an operating system with a similar design. Apple's GUI-based OS was included with the Macintosh, which was released in 1984. Microsoft released their first GUI-based OS, Windows 1.0, in 1985.
If you want to read more about Graphical Use Interface than go to the this link
Python Libraries To Create Graphical User Interfaces
Top python Libraries which is use by most of GUI developer is:
Kivy
Python
QTwxPython
Tkinter
To read basic about these Libraries first we know about "Tkinter".
What is Tkinter?
Tkinter is an inbuilt Python libraries used to create simple GUI apps. It is the most commonly used module for GUI apps in the Python.
To understand it clearly first we will see working of small code in which we will print hello world:
Example:
"""Hello World application for Tkinter"""
from tkinter import *
from tkinter.ttk import *
root = Tk()
label = Label(root, text="Hello World")
label.pack()
root.mainloop()
First line used to import module tkinter from python library
In second line tkinter.ttk used to import ttk library with tkinter to add extra features.
pack() used to pack the content so user can see it.
The last line calls the mainloop function. This function calls the endless loop of the window, so the window will wait for any user interaction till we close it.
Tkinter Widgets
Widgets are something like elements. There are different types of widgets which used in Tkinter
Label Widget
Button Widget
Entry Widget
Radiobutton Widget
Radiobutton Widget (Alternate)
Checkbutton Widget
Scale Widget: Horizontal
Scale Widget: Vertical
Text Widget
LabelFrame Widget
Canvas Widget
Listbox Widget
Menu Widget
OptionMenu Widget
Here below we discuss complete details about all widgets- how will use it and how it works?
Label Widget
A Label widget shows text to the user. Labels are used to create texts and images and all of that:
Example:
import tkinter
parent_widget = tkinter.Tk()
label_widget = tkinter.Label(parent_widget, text="A Label")
label_widget.pack()
tkinter.mainloop()
Output:
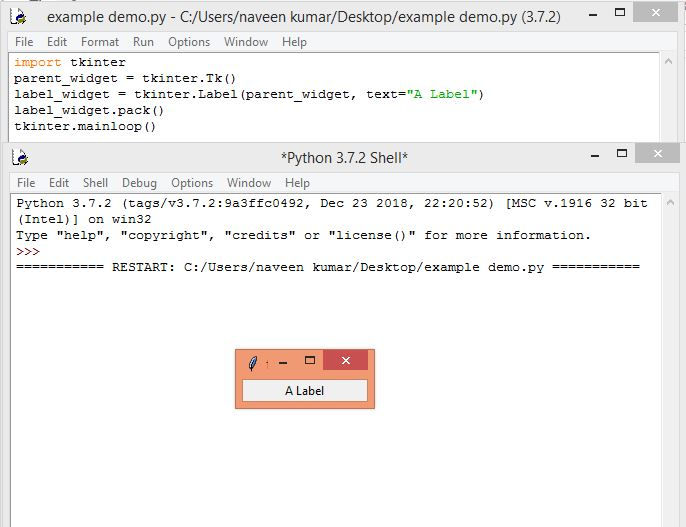
Button Widget
To add a button in your application, this widget is used.
Example:
import tkinter
window = tkinter.Tk()
button_widget = tkinter.Button(window,text="A Button")
button_widget.pack()
tkinter.mainloop()
Output:
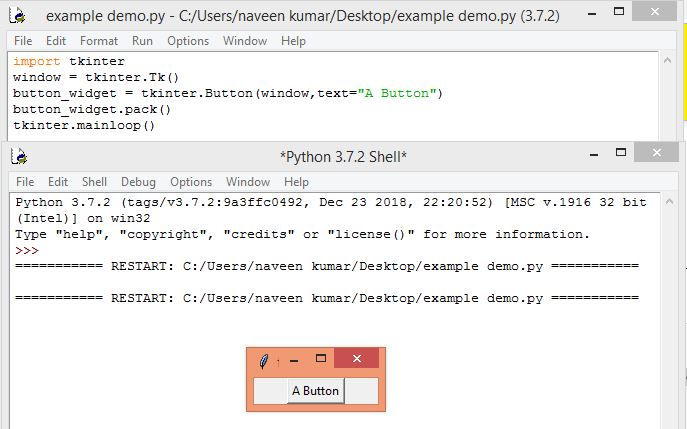
Entry Widget
An Entry widget gets text input from the user.
Example:
import tkinter
window = tkinter.Tk()
entry_widget = tkinter.Entry(window)
entry_widget.insert(0, "Type your text here")
entry_widget.pack()
tkinter.mainloop()
Output:
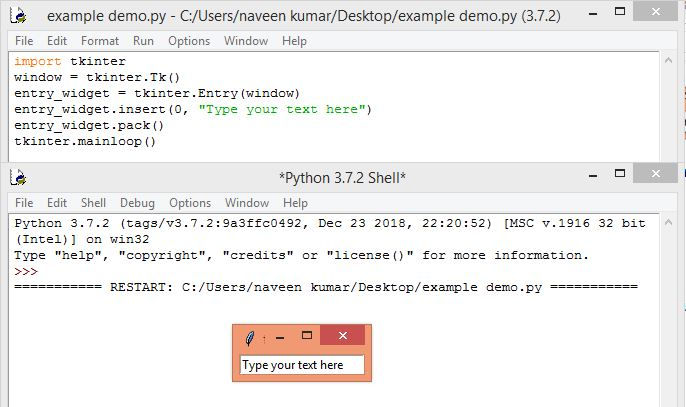
Radio button Widget
A Radiobutton lets you put buttons together, so that only one of them can be clicked. If one button is on and the user clicks another, the first is set to off.
Example:
import tkinter
window = tkinter.Tk()
v = tkinter.IntVar()
v.set(1) # need to use v.set and v.get to set and get the value of this variable
radiobutton_widget1 = tkinter.Radiobutton(window,text="Radiobutton 1",variable=v,value=1)
radiobutton_widget2 = tkinter.Radiobutton(window,text="Radiobutton 2",variable=v, value=2)
radiobutton_widget1.pack()
radiobutton_widget2.pack()
tkinter.mainloop()
Output:
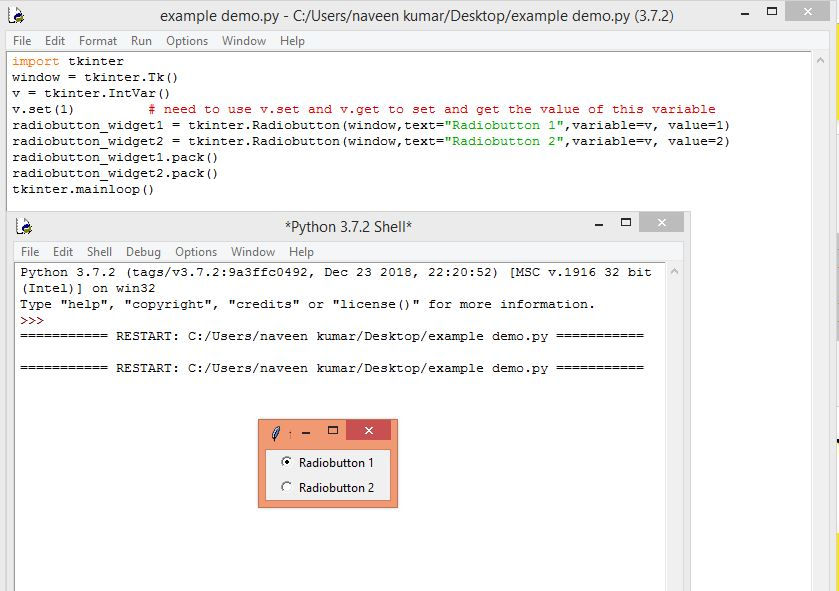
Radiobutton Widget -Alternate
You can display a Radiobutton without the dot indicator
Example:
import tkinter
window = tkinter.Tk()
v = tkinter.IntVar()
v.set(1)
radiobutton_widget1 = tkinter.Radiobutton(window,
text="Radiobutton 1",
variable=v, value=1,
indicatoron=False)
radiobutton_widget2 = tkinter.Radiobutton(window,
text="Radiobutton 2",
variable=v, value=2,
indicatoron=False)
radiobutton_widget1.pack()
radiobutton_widget2.pack()
tkinter.mainloop()
Output:
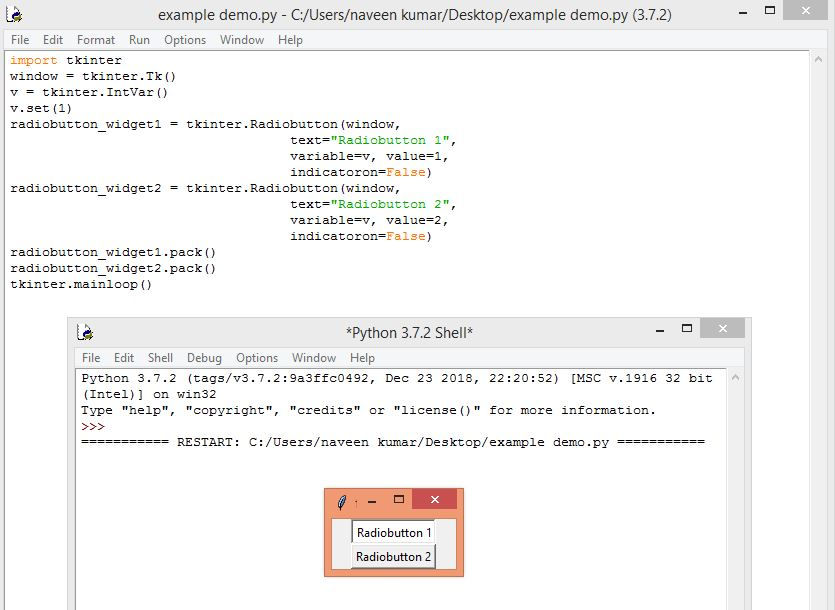
Checkbutton Widget
A Checkbutton records on/off or true/false status
Example:
import tkinter
window = tkinter.Tk()
checkbutton_widget = tkinter.Checkbutton(window,
text="Checkbutton")
checkbutton_widget.select()
checkbutton_widget.pack()
tkinter.mainloop()
Output:
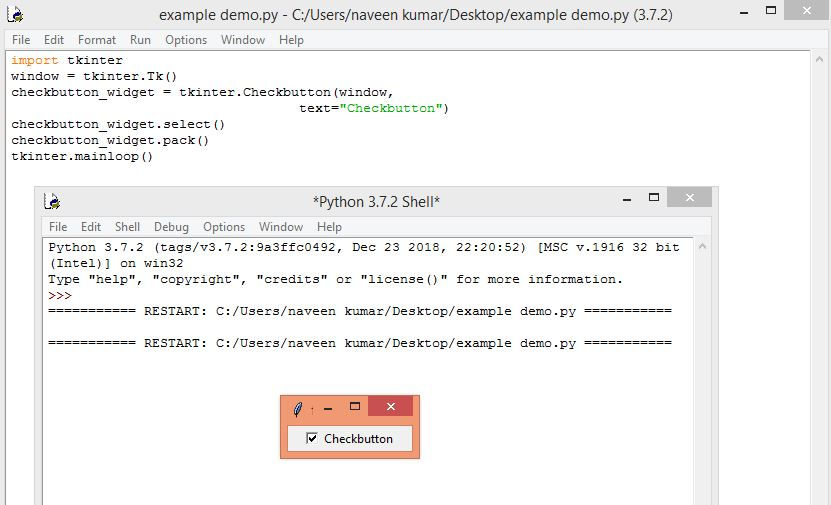
Scale Widget: Horizontal
Use a Scale widget when you want a slider that goes from one value to another.
Example:
import tkinter
window = tkinter.Tk()
scale_widget = tkinter.Scale(window, from_=0, to=100,
orient=tkinter.HORIZONTAL)
scale_widget.set(25)
scale_widget.pack()
tkinter.mainloop()
Output:
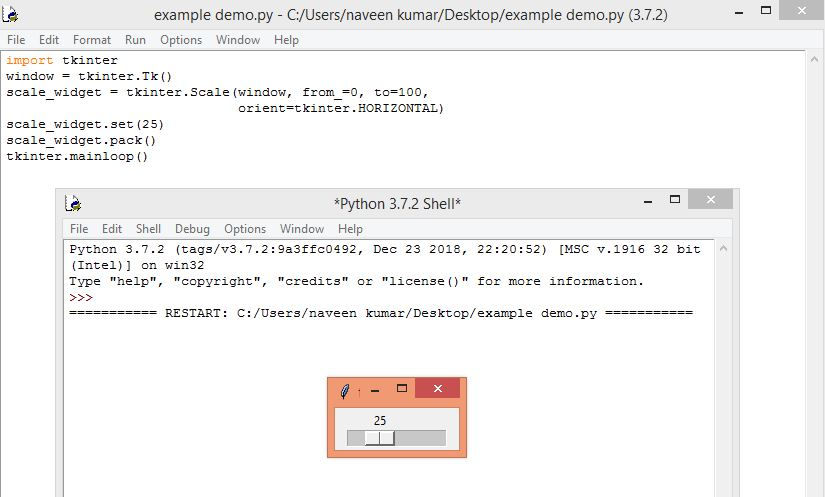
Scale Widget: Vertical
A Scale widget can be vertical (up and down).
Example:
import tkinter
window = tkinter.Tk()
scale_widget = tkinter.Scale(window, from_=0, to=100,
orient=tkinter.VERTICAL)
scale_widget.set(25)
scale_widget.pack()
tkinter.mainloop()
Output:
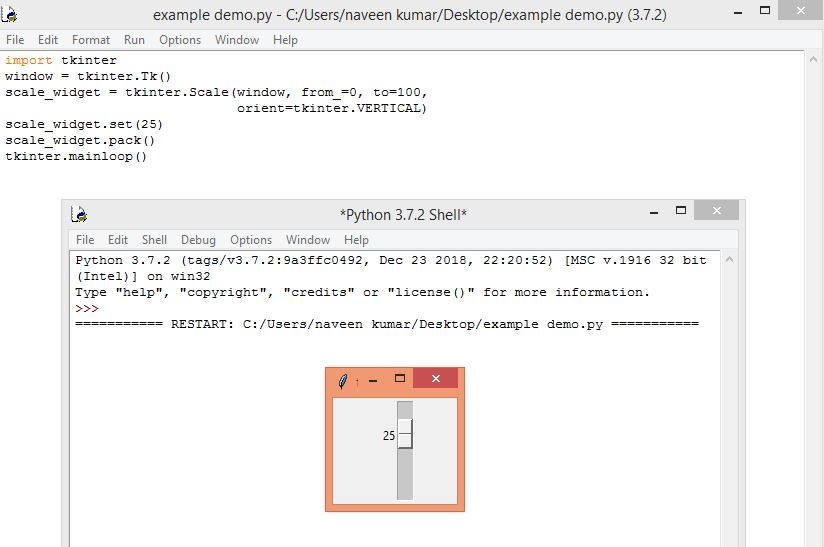
Text Widget in Python Tkinter
Use to show large areas of text.
Example:
import tkinter
window = tkinter.Tk()
text_widget = tkinter.Text(window,
width=20, height=3)
text_widget.insert(tkinter.END,
"Text Widgetn20 characters widen3 lines high")
text_widget.pack()
tkinter.mainloop()
Output:

LabelFrame Widget
The LabelFrame acts as a parent widget for other widgets, displaying them with a title and an outline. LabelFrame has to have a child widget before you can see it.
Example:
import tkinter
window = tkinter.Tk()
labelframe_widget = tkinter.LabelFrame(window,
text="LabelFrame")
label_widget=tkinter.Label(labelframe_widget,
text="Child widget of the LabelFrame")
labelframe_widget.pack(padx=10, pady=10)
label_widget.pack()
tkinter.mainloop()
Output:
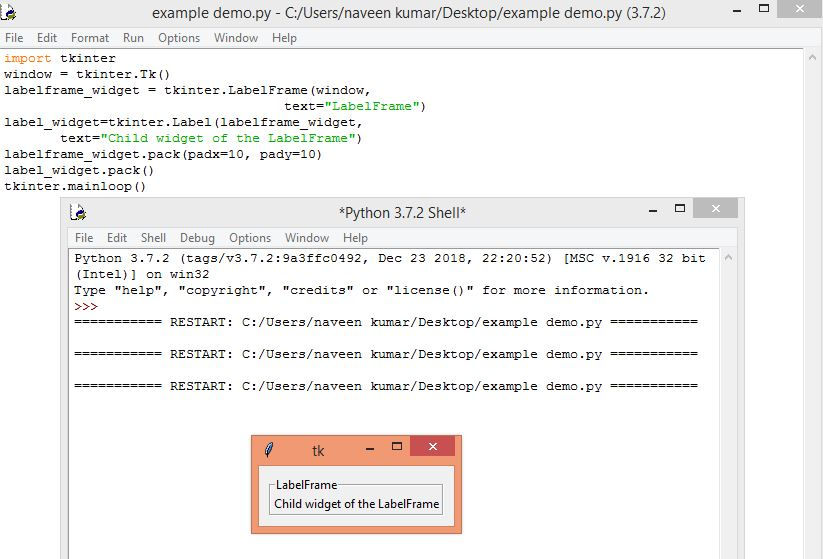
Canvas Widget
You use a Canvas widget to draw on. It supports different drawing methods.
Example:
import tkinter
window = tkinter.Tk()
canvas_widget = tkinter.Canvas(window,bg="blue",width=100,height= 50)
canvas_widget.pack()
tkinter.mainloop()
Output:
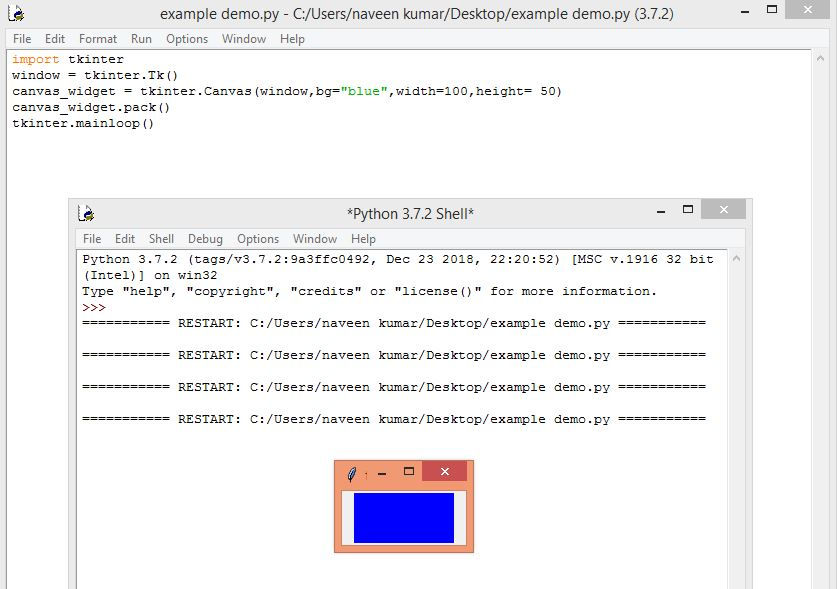
Listbox Widget
Listbox lets the user choose from one set of options or displays a list of items.
Example:
import tkinter
window = tkinter.Tk()
listbox_entries = ["Entry 1", "Entry 2",
"Entry 3", "Entry 4"]
listbox_widget = tkinter.Listbox(window)
for entry in listbox_entries:
listbox_widget.insert(tkinter.END, entry)
listbox_widget.pack()
tkinter.mainloop()
Output:
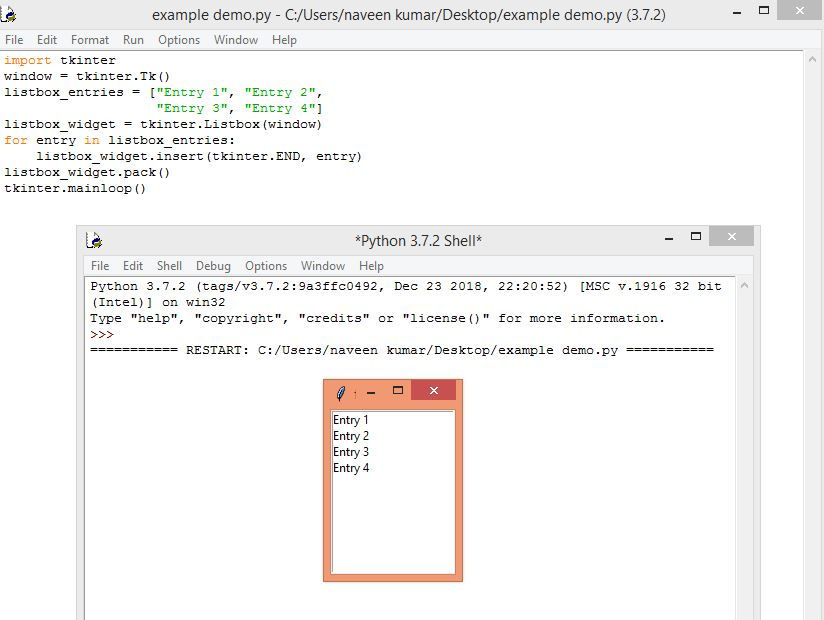
Menu Widget
The Menu widget can create a menu bar.
Example:
import tkinter
window = tkinter.Tk()
def menu_callback():
print("I'm in the menu callback!")
def submenu_callback():
print("I'm in the submenu callback!")
menu_widget = tkinter.Menu(window)
submenu_widget = tkinter.Menu(menu_widget, tearoff=False)
submenu_widget.add_command(label="Submenu Item1",
command=submenu_callback)
submenu_widget.add_command(label="Submenu Item2",
command=submenu_callback)
menu_widget.add_cascade(label="Item1", menu=submenu_widget)
menu_widget.add_command(label="Item2",
command=menu_callback)
menu_widget.add_command(label="Item3",
command=menu_callback)
window.config(menu=menu_widget)
tkinter.mainloop()
Output:
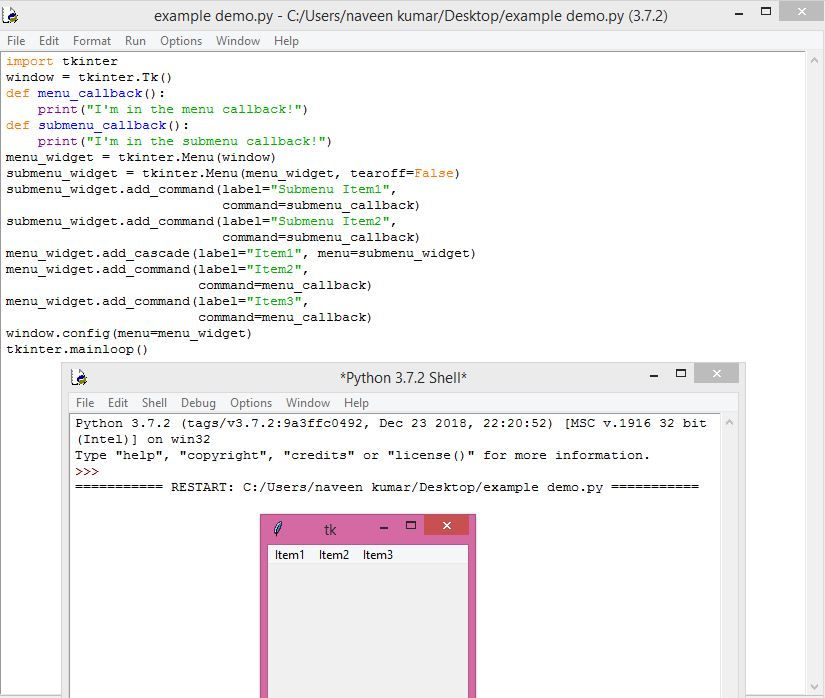
OptionMenu Widget
The OptionMenu widget lets the user choose from a list of options
Example:
import tkinter
window = tkinter.Tk()
control_variable = tkinter.StringVar(window)
OPTION_TUPLE = ("Option 1", "Option 2", "Option 3")
optionmenu_widget = tkinter.OptionMenu(window,control_variable, *OPTION_TUPLE)
optionmenu_widget.pack()
tkinter.mainloop()
Output:
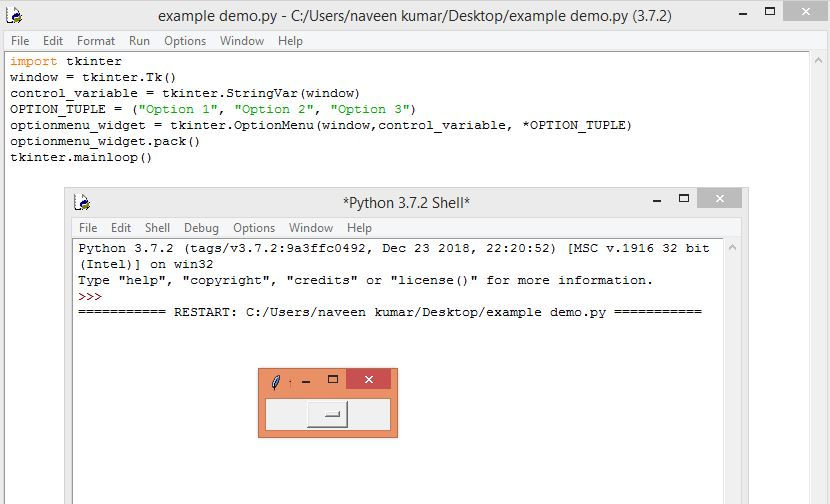
Geometry Management in Python
Tkinter possess three layout managers.
pack
grid
place
Example
import tkinter as tk
root = tk.Tk()
w = tk.Label(root, text="Red Sun", bg="red", fg="white")
w.pack(fill=tk.X)
w = tk.Label(root, text="Green Grass", bg="green", fg="black")
w.pack(fill=tk.X)
w = tk.Label(root, text="Blue Sky", bg="blue", fg="white")
w.pack(fill=tk.X)
tk.mainloop()
Output:

Binding Function in python
It used to bind the event by mouse or keyboard to perform specific action
Example:
#!/usr/bin/python3
# write tkinter as Tkinter to be Python 2.x compatible
from tkinter import *
def hello(event):
print("Single Click, Button-l")
def quit(event):
print("Double Click, so let's stop")
import sys; sys.exit()
widget = Button(None, text='Mouse Clicks')
widget.pack()
widget.bind('<Button-1>', hello)
widget.bind('<Double-1>', quit)
widget.mainloop()
Output:
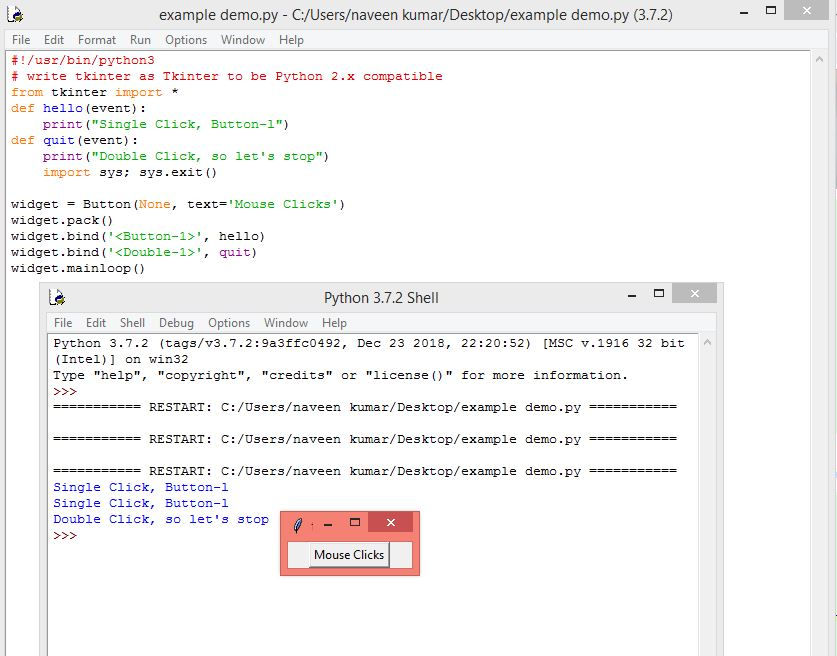
List of Other Codersarts Assignment Expert Help Services
If you like Codersarts blog and looking for Programming Assignment Help Service,Database Development Service,Web development service,Mobile App Development, Project help, Hire Software Developer,Programming tutors help and suggestion you can send mail at contact@codersarts.com.
Please write your suggestion in comment section below if you find anything incorrect in this blog post
Comments