
Introduction to Gradio
Gradio is a powerful Python library that has been gaining traction in the machine learning and deep learning communities for its ability to simplify the development of interactive and user-friendly machine learning applications. Whether you are a data scientist or a developer with a keen interest in AI, Gradio can significantly ease the process of building and deploying machine learning models.
Gradio acts as a bridge between your machine learning model and the end user, allowing you to create intuitive user interfaces for your models with minimal effort. What makes Gradio particularly appealing is its versatility—it supports a wide range of input and output types, making it suitable for a variety of machine learning tasks, from image classification and text generation to natural language processing and more.
This blog post will teach you everything you need to know about Gradio, including why it's important for machine learning and how to use it to create your own interactive applications. We'll cover everything from installation and setup to customization, deployment options, real-world use cases, and more. By the end of this journey, you'll have a solid understanding of why Gradio has become an indispensable tool for machine learning practitioners and developers alike.
Let's learn about Gradio and see how it can change the way you use machine learning models.
Installation and Setup
Before we dive deeper into the world of Gradio, let's start with the basics—how to install and set up the library. Gradio strives to be accessible to all users, and its installation process is no exception. You can quickly get Gradio up and running on your system in just a few steps.
Using pip
If you're using pip, open your terminal or anaconda command prompt and execute the following command:
pip install gradio
Using conda
For those who prefer conda, you can install Gradio with the following command:
conda install -c conda-forge gradio
Once the installation is complete, you're ready to start building interactive machine learning applications with Gradio.
Key Features of Gradio
Now that we've got Gradio installed, let's take a closer look at some of the key features that make Gradio a standout library for building interactive machine learning applications.
1. Simplicity and Ease of Use:
Gradio is designed with simplicity in mind. It abstracts away much of the complexity involved in creating user interfaces for machine learning models. With Gradio, you can focus on your model's core logic and let the library handle the user interface.
2. Support for Various Input and Output Types:
Gradio supports a wide range of input types, including text, images, audio, and video. It also accommodates various output types, such as text, images, and plots. This flexibility makes it suitable for a diverse set of machine learning tasks.
3. Compatibility with Popular Deep Learning Frameworks:
Gradio seamlessly integrates with popular deep learning frameworks like TensorFlow, PyTorch, and scikit-learn. This means you can easily incorporate your pre-trained models into Gradio applications.
4. Real-time Updates:
Gradio provides real-time updates, allowing your machine learning model to make predictions as the user interacts with the interface. This feature is especially valuable for applications like image classification, where users can see predictions as they upload images.
5. Interactivity and Customization:
Gradio enables you to add interactivity to your models. Users can input data and receive immediate feedback, enhancing the user experience. Furthermore, you can customize the appearance and behavior of your interfaces to match your application's requirements.
6. Scalability and Performance:
Gradio is built to handle concurrent requests efficiently, making it suitable for both small-scale and large-scale deployments. It also has features for caching and optimizing performance, ensuring a smooth user experience even with heavy usage.
7. Community and Open Source:
Gradio benefits from an active open-source community. You can find tutorials, documentation, and examples online. Additionally, the library is constantly evolving, with updates and improvements driven by user feedback and contributions.
Interactive Image Classification with Gradio and TensorFlow
In this section, we'll demonstrate how to create an interactive image classification application using Gradio and TensorFlow. We'll use a pre-trained MobileNetV2 model to classify user-uploaded images into the top three categories.
Setting up the Environment
To get started, we need to set up the necessary environment. We'll load the MobileNetV2 model and download human-readable labels for ImageNet. This will allow us to map the model's predictions to meaningful class labels.
#import library
import tensorflow as tf
import requests
import gradio as gr
# Load the InceptionNet model
inception_net = tf.keras.applications.MobileNetV2()
# Download human-readable labels for ImageNet
response = requests.get("https://git.io/JJkYN")
labels = response.text.split("\n")
Defining the Classification Function
Next, we define a function called classify_image that takes an image as input and returns the top three predicted categories along with their confidence scores.
# Define the function to classify an image
def classify_image(image):
# Preprocess the user-uploaded image
image = image.reshape((-1, 224, 224, 3))
image = tf.keras.applications.mobilenet_v2.preprocess_input(image)
# Make predictions using the MobileNetV2 model
prediction = inception_net.predict(image).flatten()
# Get the top 3 predicted labels with their confidence scores
top_classes = [labels[i] for i in prediction.argsort()[-3:][::-1]]
top_scores = [float(prediction[i]) for i in prediction.argsort()[-3:][::-1]]
return {top_classes[i]: top_scores[i] for i in range(3)}
Creating the Gradio Interface
With the classification function in place, we can now create the Gradio interface. This interface allows users to upload an image, and the model will classify it into the top three categories.
# Create the Gradio interface
iface = gr.Interface(
fn=classify_image,
inputs=gr.Image(shape=(224, 224)),
outputs=gr.Label(num_top_classes=3),
live=True,
capture_session=True, # This captures the user's uploaded image
title="Image Classification",
description="Upload an image, and the model will classify it into the top 3 categories.",
)
Launching the Gradio Interface
Finally, we launch the Gradio interface, making our image classification application accessible to users.
# Launch the Gradio interface
iface.launch()
Now, when users access this interface, they can upload an image, and the model will provide real-time predictions as shown below, displaying the top three categories along with their confidence scores.
Output
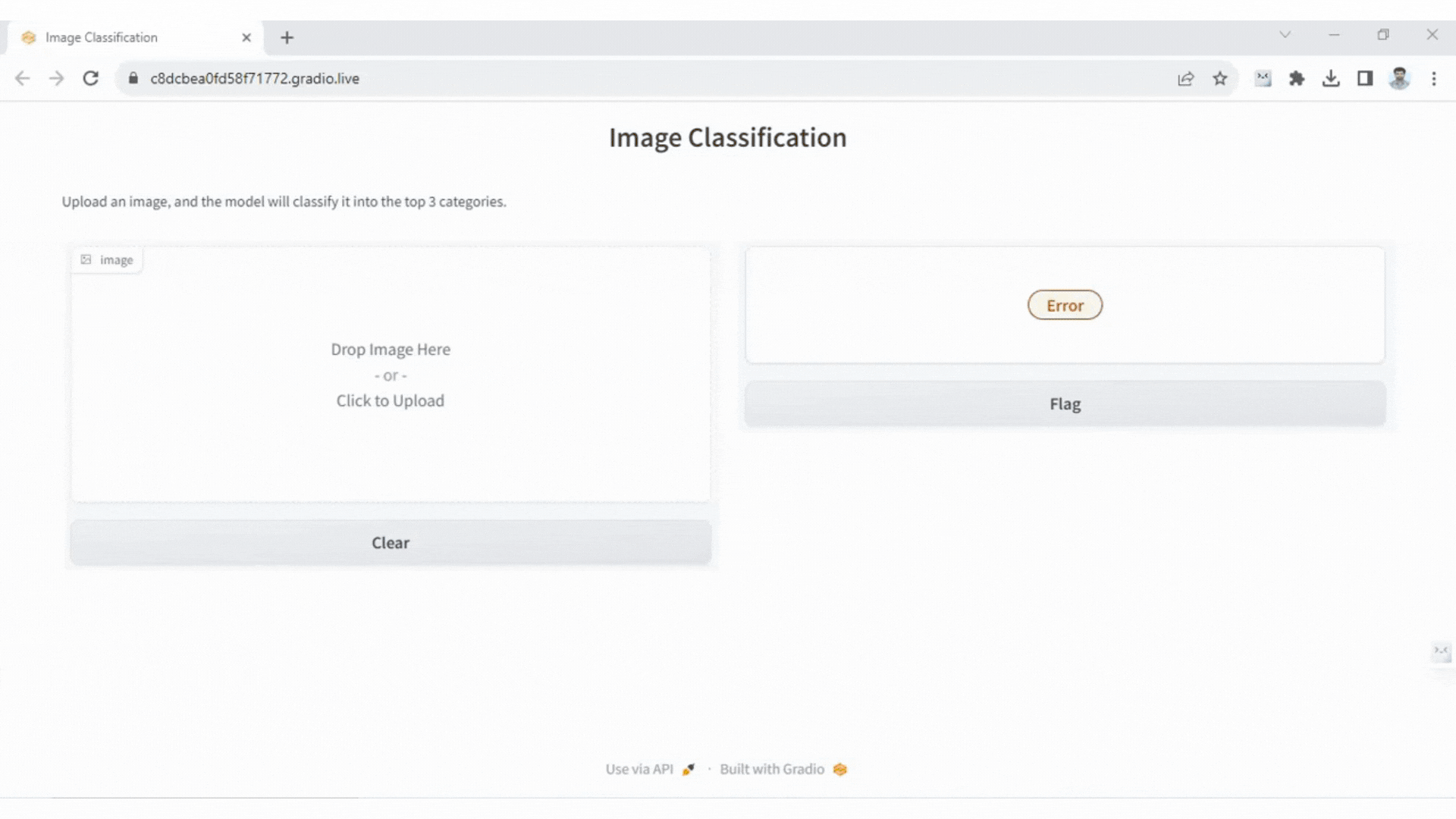
Interactive Demos with Gradio
Gradio shines not only as a tool for building interactive machine learning applications but also as a platform for creating engaging and informative demos. In this section, we'll explore how Gradio simplifies the process of showcasing machine learning models to a broader audience through interactive demos.
Bringing Models to Life
One of the most compelling ways to convey the capabilities of a machine learning model is by allowing users to interact with it directly. Gradio enables you to transform a static model into an interactive tool that users can experiment with in real-time. Whether it's image classification, text generation, or any other machine learning task, Gradio's interactivity feature breathes life into your models.
Example: Image Captioning Demo
Imagine you have a trained image captioning model, and you want to showcase its abilities to generate captions for user-uploaded images. With Gradio, you can easily create an interactive demo for this purpose:
# import library
import gradio as gr
# Define the image captioning function
def generate_caption(image):
caption = model.generate_caption(image)
return caption
# Create the Gradio interface
iface = gr.Interface(
fn=generate_caption,
inputs=gr.Image(shape=(224, 224)),
outputs=gr.Textbox(),
live=True,
title="Image Captioning Demo",
description="Upload an image, and the model will generate a caption for it.",
)
# Launch the Gradio interface
iface.launch()
Customization: Tailoring Gradio Interfaces to Your Needs
Gradio not only simplifies the process of creating machine learning interfaces but also empowers users with the ability to customize these interfaces to match their specific requirements. Let's explore how Gradio allows for extensive customization in terms of appearance and behavior.
Layout Customization
One of the primary ways you can customize a Gradio interface is by adjusting its layout. Gradio provides several layout options, allowing you to control the arrangement of input and output components on the interface. This feature is especially valuable when designing intuitive and user-friendly interfaces for your machine learning models.
# Example of layout customization
iface = gr.Interface(
fn=classify_image,
inputs=["text", "image"],
outputs="text",
layout="horizontal",
title="Custom Layout",
description="This interface has a custom horizontal layout.",
).launch()
In the code snippet above, we've set the layout to "horizontal," which arranges the input and output components side by side. Gradio supports other layout options like "vertical," "grid," and "sidebar," allowing you to choose the one that best suits your application's design.
Output

Adding Descriptions and Labels
To provide context and guidance to users, you can add descriptions and labels to your Gradio interface components. This makes the interface more informative and user-friendly. For instance, you can include explanations for input fields or labels for output predictions.
# Example of adding descriptions and labels
iface = gr.Interface(
fn=classify_image,
inputs=gr.Textbox(label="Enter text:", placeholder="Type here..."),
outputs=gr.Label(label="Prediction:"),
title="Customized Interface",
description="This interface has custom labels and descriptions.",
).launch()
In the above code, we've added labels like "Enter text:" and "Prediction:" to provide clear instructions to users. Descriptive labels can make the interface more intuitive and help users understand the purpose of each component.
Output

Using Pre-built Components
Gradio offers a range of pre-built input and output components that you can leverage to enhance your interface. These components are designed to handle various types of data, from text and images to audio and video. By using these components, you can quickly create interactive interfaces without the need for extensive custom coding.
# Example of using pre-built components
iface = gr.Interface(
fn=classify_image,
inputs=gr.Textbox(),
outputs=gr.Label(),
title="Using Pre-built Components",
description="This interface uses pre-built components for input and output.",
).launch()
Output

Deployment Options: Sharing Your Gradio
Applications with the World
Building interactive machine learning applications with Gradio is just the beginning. To truly make your models accessible and useful, you need to deploy them where users can interact with them. Gradio offers several deployment options, making it easy to share your applications with a global audience.
Local Deployment
For development and testing purposes, you can deploy your Gradio applications locally on your own machine. This is a convenient way to ensure everything is working as expected before sharing your application with others.
# Local deployment example
iface.launch()
By calling the launch() method without any arguments, Gradio will deploy your application locally, typically on http://localhost:7860. Users can access the interface by opening a web browser on the same machine.
Cloud Deployment
To make your Gradio applications accessible to users worldwide, you can deploy them to cloud platforms. Gradio supports popular cloud services like Heroku, AWS, and Google Cloud. Deployment to the cloud allows you to share your machine learning models without the need for users to install any software or set up local environments.
The exact deployment process may vary depending on the cloud platform you choose, but Gradio's flexibility ensures that you can adapt your applications for cloud deployment seamlessly.
Shareable URLs
Gradio simplifies sharing your machine learning interfaces by providing shareable URLs. When you deploy your application, Gradio generates a unique URL that you can distribute to users. They can access your application directly through their web browsers without any installations or configurations.
# Example of generating a shareable URL
shareable_url = iface.share()
print(f"Share this URL: {shareable_url}")
This shareable URL can be easily shared via email, social media, or any communication channel of your choice.
Embedding in Websites
Gradio also allows you to embed your machine learning interfaces within existing websites or web applications. This feature is particularly useful if you have an established web presence and want to integrate machine learning capabilities seamlessly.
# Example of embedding Gradio in a website
html_code = iface.launch(share=True)
By including the share=True parameter when launching your interface, Gradio provides HTML code that you can embed in your website, giving users access to your machine learning features without leaving your site.
Integration with APIs
Gradio interfaces can be integrated with APIs, making it possible to incorporate machine learning capabilities into larger applications or services. This is a powerful way to extend the reach and functionality of your models by allowing other developers to interact programmatically with your Gradio application.
# Example of integrating Gradio with an API
gr.Interface.fn_to_interface(your_function).launch(share=True)
Gradio makes it straightforward to expose your machine learning models as APIs, enabling other developers to leverage your models in their own applications.
Conclusion
In conclusion, Gradio is a remarkable tool that empowers both developers and data scientists to effortlessly create interactive machine learning applications. Its versatility, ease of use, and customization options make it a valuable asset in bridging the gap between complex models and end users. Whether you aim to deploy locally or in the cloud, share interfaces via URLs, or embed them in websites, Gradio provides the flexibility needed to make machine learning accessible and impactful. As you explore the endless possibilities of Gradio, remember that innovation in AI and machine learning is at your fingertips, waiting to be harnessed to create user-friendly, interactive solutions that can transform the way we interact with AI models.
