
Introduction to Streamlit
Streamlit is a Python library that has taken the world of data science and web application development by storm. Its rise in popularity can be attributed to its simplicity and effectiveness in creating interactive web applications with minimal effort. Whether you're a data scientist, machine learning engineer, or a developer looking to showcase your work, Streamlit offers a streamlined solution to turn your Python scripts into fully functional web apps.
At its core, Streamlit is designed to bridge the gap between data analysis and web application deployment. It allows you to transform your data-driven Python code into web-based interfaces that are not only user-friendly but also highly customizable. What sets Streamlit apart is its remarkable ease of use, making it accessible to individuals with varying levels of programming experience.
In essence, Streamlit democratizes the process of creating web applications. You don't need to be a web development expert to build a web app that displays your data or machine learning models. With just a few lines of Python code, you can create interactive dashboards, data visualizations, and machine learning tools that are both functional and visually appealing.
Streamlit's intuitive API and built-in widgets enable you to focus on your data and the logic of your application, rather than getting bogged down in the complexities of web development. Whether you're a beginner exploring the world of data science or a seasoned developer looking for a rapid development framework, Streamlit is a powerful tool in your arsenal.
In this blog post, we will dive deeper into the reasons why Streamlit is so relevant in today's data-driven landscape. We'll explore its ease of use, its capabilities for data visualization and machine learning integration, customization options, deployment possibilities, and much more. By the end of this journey, you'll have a comprehensive understanding of why Streamlit has become a must-know library for anyone working with data and looking to share their insights with the world.
So, let's embark on this exploration of Streamlit's relevance and discover how it can empower you to turn your data-driven ideas into interactive web applications effortlessly.
Ease of Use
One of Streamlit's most compelling features is its unparalleled ease of use. It is engineered to simplify the process of creating web applications to the point where even those with limited programming experience can quickly become proficient app developers.
Python-Centric Development
Streamlit is designed to work seamlessly with Python, a language known for its readability and ease of learning. This means you don't need to learn multiple languages or complex frameworks to build web apps. You can leverage your existing Python skills and libraries to create interactive applications.
Minimal Code Requirements
With just a few lines of Python code, you can have a functional web application up and running. Streamlit abstracts away many of the complexities associated with web development, such as setting up server routes, handling HTTP requests, or writing HTML/CSS. This streamlined approach allows you to focus on your data and application logic.
Here's a quick example of how easy it is to create a basic Streamlit app:
#import library
import streamlit as st
# Create a title for your app
st.title("My First Streamlit App")
# Add content to your app
st.write("Hello, World!")
# Display a chart
import pandas as pd
import numpy as np
chart_data = pd.DataFrame(np.random.randn(20, 3), columns=["a", "b", "c"])
st.line_chart(chart_data)
This simple script creates a web application with a title, some text, and a line chart. Streamlit takes care of turning this code into a functional web app that can be accessed through a web browser.
Output

Interactive Widgets
Streamlit provides a wide range of interactive widgets, such as sliders, buttons, and text input fields, which can be easily added to your app. These widgets allow users to interact with your data and customize their experience. For instance, you can create a data exploration tool with sliders to adjust parameters or filters to refine data views.
# Add a slider widget
x = st.slider('Select a value', 0, 100)
# Show the selected value
st.write(f'You selected: {x}')
These widgets require only a single line of code to implement, further demonstrating Streamlit's simplicity.
Output

Instant Feedback
One of Streamlit's remarkable features is its instant feedback loop. As you make changes to your code, you can see the updates in real-time in your web app. This iterative development process greatly accelerates the creation of web applications, as you can quickly experiment and refine your app's user interface and functionality.
Streamlit's ease of use is a fundamental reason for its popularity. Whether you're a data scientist, researcher, or developer, you can leverage Streamlit to build web applications without the steep learning curve typically associated with web development. This accessibility makes it an invaluable tool for turning your data projects into interactive, shareable applications.
Data Visualization
Streamlit isn't just about creating simple web interfaces; it's a powerful tool for crafting compelling data visualizations that make your insights more accessible and engaging. Here's how Streamlit excels in this aspect:
Seamless Integration with Data Visualization Libraries
Streamlit seamlessly integrates with popular data visualization libraries like Matplotlib, Plotly, Altair, and others. This means you can leverage the full capabilities of these libraries to create stunning charts, graphs, and interactive plots directly within your Streamlit applications.
For example, you can use Matplotlib to generate custom visualizations and then display them effortlessly in your Streamlit app:
import streamlit as st
import matplotlib.pyplot as plt
import numpy as np
# Create a figure using Matplotlib
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
ax.plot(x, y)
# Display the Matplotlib figure in Streamlit
st.pyplot(fig)
This combination of Streamlit and data visualization libraries empowers you to create dynamic and informative charts that communicate your data-driven insights effectively.
Output

Interactive Dashboards
With Streamlit's interactive widgets, you can transform static plots into dynamic dashboards. Users can tweak parameters, filter data, or select different datasets, allowing for real-time exploration of your visualizations. This level of interactivity is invaluable when you want to empower users to gain deeper insights from your data.
# import libraries
import streamlit as st
import pandas as pd
# Create a DataFrame
data = pd.read_csv('data.csv')
# Add a dropdown widget for selecting a column to visualize
selected_column = st.selectbox('Select a column:', data.columns)
# Create a chart based on the selected column
st.line_chart(data[selected_column])
Output
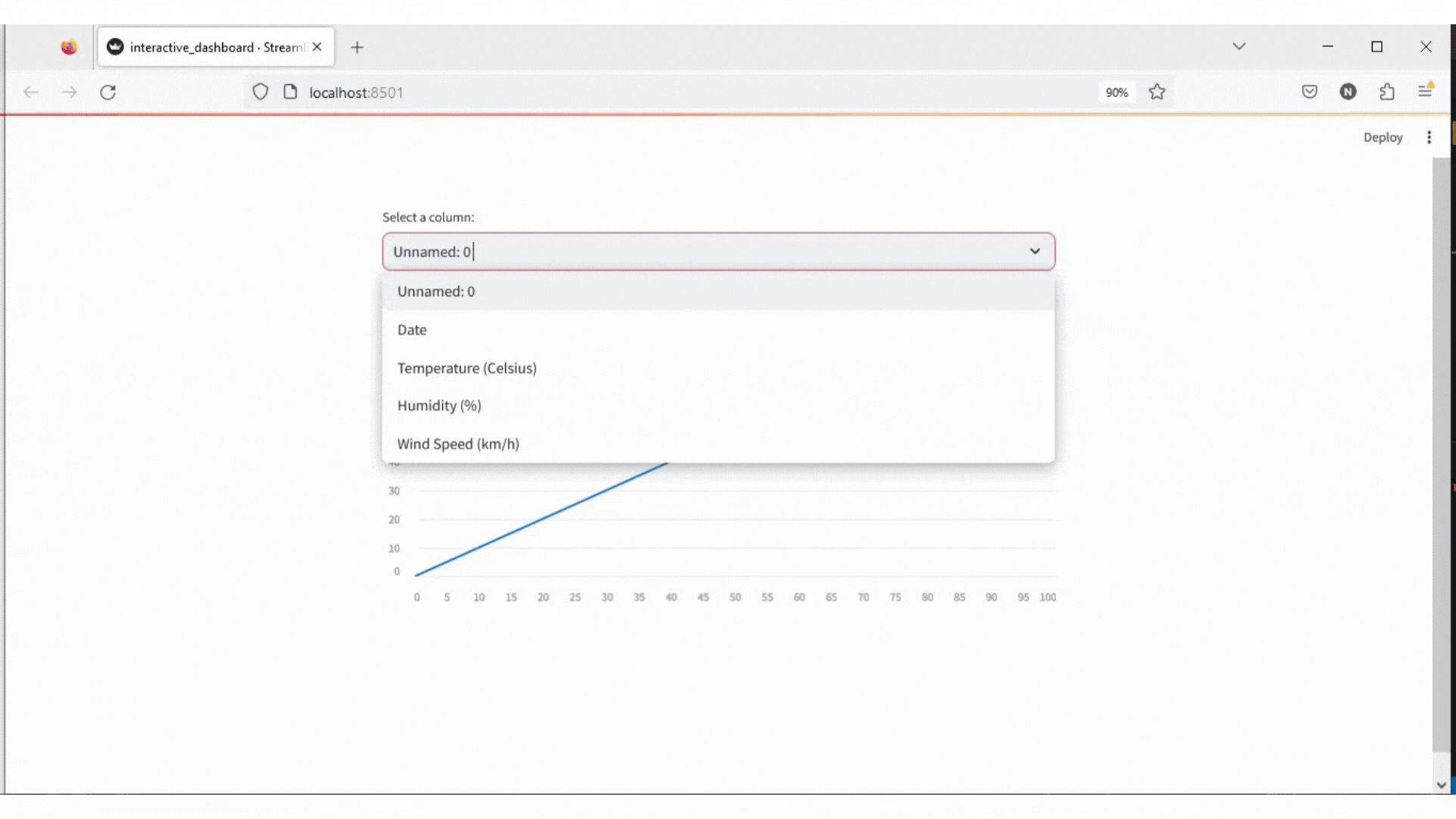
Sharing Insights
Whether you're presenting your findings to colleagues, clients, or the general public, Streamlit makes it easy to share your data-driven insights. You can publish your Streamlit app on various platforms, making it accessible to a wide audience. This can be particularly useful when you want to communicate complex data analyses or machine learning models to stakeholders who may not have technical backgrounds.
Real-Time Data Updates
Streamlit apps can be designed to update in real-time as your data changes. For example, if you're monitoring live data streams or updating your datasets regularly, you can configure your Streamlit app to refresh and display the latest information automatically.
Streamlit's ability to seamlessly integrate with data visualization libraries, provide interactive dashboards, facilitate the sharing of insights, and support real-time updates makes it a versatile choice for anyone looking to convey data-driven stories effectively. Whether you're a data analyst, scientist, or a business professional, Streamlit empowers you to create visually compelling and interactive data presentations with ease.
Integration with Machine Learning
Streamlit is not limited to data visualization; it also seamlessly integrates with machine learning libraries, making it a valuable tool for building interactive machine learning applications and dashboards. Here's how Streamlit can be your go-to choice for ML integration:
Streamlined Model Deployment
One of the challenges in machine learning is deploying models into production. Streamlit simplifies this process by allowing you to wrap your machine learning models in a user-friendly web interface. You can create apps that take user inputs, pass them through your models, and display the results—all with just a few lines of code.
import streamlit as st
import pandas as pd
from sklearn.datasets import load_iris
from sklearn.ensemble import RandomForestClassifier
import joblib
# Load the Iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Train a Random Forest model
model = RandomForestClassifier()
model.fit(X, y)
# Save the trained model to a file (model.pkl)
joblib.dump(model, 'model.pkl')
# Streamlit App
st.title('Iris Flower Species Prediction')
# Add text input fields for user input
sepal_length = st.text_input('Enter Sepal Length (cm):')
sepal_width = st.text_input('Enter Sepal Width (cm):')
petal_length = st.text_input('Enter Petal Length (cm):')
petal_width = st.text_input('Enter Petal Width (cm):')
# Load the trained model
loaded_model = joblib.load('model.pkl')
# Check if all input fields are filled
if sepal_length and sepal_width and petal_length and petal_width:
# Make predictions based on user input
user_input = [[float(sepal_length), float(sepal_width), float(petal_length), float(petal_width)]]
prediction = loaded_model.predict(user_input)[0]
predicted_species = iris.target_names[prediction]
# Display the predicted species
st.write(f'Predicted Species: {predicted_species}')
else:
st.write('Please enter values in all input fields to make a prediction.')
This code example demonstrates how to load a machine learning model and use Streamlit to create a simple text classification app. The user can input text, and the model predicts a category.
Output
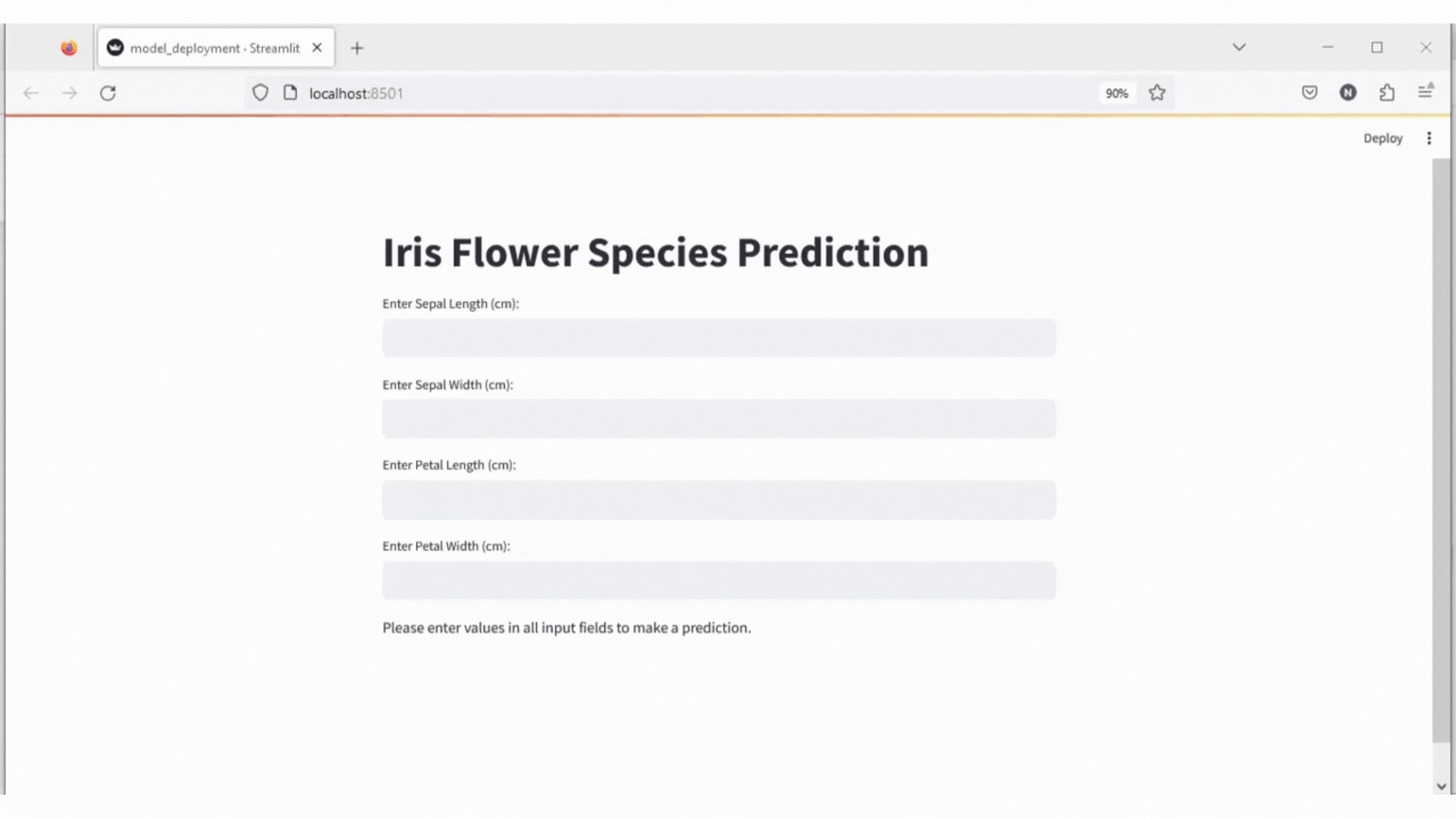
Interactive Model Tuning
Streamlit's widgets come in handy when you want to allow users to fine-tune model parameters. Whether it's adjusting hyperparameters, selecting features, or setting thresholds, you can create interactive sliders, dropdowns, and input fields to give users control over the model's behavior.
# import library
import streamlit as st
# Add a slider widget for adjusting a hyperparameter
learning_rate = st.slider('Learning Rate', 0.01, 1.0, 0.1)
# Show the selected learning rate
st.write(f'Selected Learning Rate: {learning_rate}')
This interactivity enables users to experiment with different settings and see the immediate impact on model performance.
Output

Visualizing Model Outputs
In machine learning, it's crucial to not only provide predictions but also to explain model decisions. Streamlit makes it easy to visualize the output of your models, whether it's a classification probability distribution, feature importance scores, or any other relevant information. You can display these visualizations alongside your predictions, making it easier for users to understand and trust the model's results.
#import libraries
import streamlit as st
import matplotlib.pyplot as plt
import numpy as np
# Create a bar chart to visualize feature importance
features = ['Feature 1', 'Feature 2', 'Feature 3']
importance = np.array([0.8, 0.6, 0.4])
fig, ax = plt.subplots()
ax.bar(features, importance)
st.pyplot(fig)
Output

Real-Time Model Updates
Just like with data visualizations, Streamlit apps can update in real-time based on user interactions or changes in the underlying data. This feature is especially valuable when you want to provide live predictions, monitor model performance, or track data streams.
In summary, Streamlit's integration with machine learning libraries empowers data scientists and machine learning engineers to deploy models, create interactive dashboards, fine-tune model parameters, and explain model decisions—all within a user-friendly web interface. It bridges the gap between data science and application development, making it easier than ever to put machine learning models into the hands of end-users.
Customization
While Streamlit is known for its simplicity, it also offers a high degree of customization to tailor your web applications to your specific needs and branding. Here's how you can make your Streamlit apps unique and visually appealing:
Theming
Streamlit provides theming capabilities that allow you to change the look and feel of your applications easily. You can choose from existing themes or create your custom themes to match your organization's branding or personal style preferences.
# import library
import streamlit as st
# Change the theme to a custom theme
st.set_page_config(
page_title="My Custom Streamlit App",
page_icon=":chart_with_upwards_trend:",
layout="centered", # Choose layout options: "centered" or "wide"
)
# Add some content to the Streamlit app
st.title("Welcome to My Custom Streamlit App")
st.write("This is a simple Streamlit app with custom settings.")
st.write("You can add more content, widgets, or charts here.")
You can also customize colors, fonts, and other style elements to ensure that your Streamlit app aligns with your visual identity.
Output

Widgets and Layout
Streamlit's widgets and layout components allow you to design your app's interface exactly the way you want it. You can use layout functions like st.columns() to create multi-column layouts, control spacing, and arrange widgets precisely.
# import library
import streamlit as st
# Create a multi-column layout
col1, col2 = st.columns(2)
# Add widgets to each column
with col1:
st.header("Column 1")
st.button("Button 1")
with col2:
st.header("Column 2")
st.button("Button 2")
This flexibility enables you to design complex and visually appealing dashboards and applications.
Output

Custom CSS and HTML
For advanced users, Streamlit allows you to inject custom CSS and HTML into your applications. This level of customization gives you full control over the app's appearance and functionality.
# import library
import streamlit as st
# Add custom CSS styles
st.markdown(
"""
<style>
/* Your custom CSS styles here */
</style>
""",
unsafe_allow_html=True,
)
# Add custom HTML elements
st.markdown(
"""
<div>
<!-- Your custom HTML content here -->
</div>
""",
unsafe_allow_html=True,
)
This feature is particularly useful if you have specific design requirements or need to integrate third-party libraries and visual components.
Output

Extensions and Plugins
Streamlit's active community has developed various extensions and plugins that can enhance the functionality and appearance of your applications. These extensions cover a wide range of use cases, from interactive maps to custom data visualization components. You can easily incorporate these extensions into your Streamlit projects to extend their capabilities.
In summary, Streamlit's customization options ensure that you can create web applications that not only serve their functional purpose but also align with your brand identity and design preferences. Whether you need a simple and clean interface or a highly stylized application, Streamlit provides the tools to make your vision a reality.
Deployment Options
Streamlit's versatility extends to deployment, offering multiple options to make your web applications accessible to your target audience. Whether you want to share your work with colleagues, clients, or the general public, Streamlit provides straightforward solutions:
Self-Hosting
For those who prefer to maintain control over their web applications, Streamlit allows you to self-host your apps on your own servers or cloud infrastructure. You can deploy your Streamlit app on platforms like AWS, Google Cloud, or Azure, making it accessible through a custom domain or IP address.
Self-hosting gives you complete control over the deployment environment, ensuring that your app runs securely and efficiently. It's an excellent choice for organizations with strict data security requirements or those who want to integrate Streamlit apps into their existing infrastructure.
Streamlit Sharing
Streamlit offers a dedicated deployment platform called "Streamlit Sharing." It's a free hosting solution specifically designed for Streamlit apps. With Streamlit Sharing, you can deploy your apps quickly and share them with others via a public URL. This is a great option for showcasing your projects, creating interactive demos, or collaborating with team members.
Deploying on Streamlit Sharing is as simple as pushing your code to a GitHub repository. The platform takes care of the deployment process, ensuring that your app is live and accessible with minimal effort.
Docker Containers
If you prefer containerization, you can package your Streamlit app into a Docker container. Docker containers are portable and can be deployed on various cloud platforms and container orchestration systems like Kubernetes. This approach allows for easy scalability and management of your applications, making it suitable for large-scale deployments.
Serverless Deployment
For serverless enthusiasts, Streamlit apps can be deployed using serverless computing platforms like AWS Lambda or Google Cloud Functions. Serverless architectures automatically scale based on demand, ensuring that your app can handle varying levels of traffic efficiently. It's a cost-effective solution for apps with unpredictable usage patterns.
Sharing as a GitHub Repository
Another straightforward way to share Streamlit apps is by hosting them as part of a GitHub repository. This allows you to leverage GitHub Pages to make your app accessible online. You can create a dedicated "docs" folder in your repository and deploy your Streamlit app there. Users can access your app using a GitHub Pages URL, and you can maintain version control and collaborate with others through GitHub.
Streamlit offers a range of deployment options to suit your specific needs and preferences. Whether you want simplicity with Streamlit Sharing, control with self-hosting, scalability with containers, or cost-effectiveness with serverless deployment, Streamlit provides the flexibility to make your web applications accessible to your intended audience. This variety of options ensures that you can choose the deployment strategy that best aligns with your project's requirements.
Conclusion
Streamlit is a game-changer in the realm of data science and web application development. Its simplicity, seamless integration with data visualization and machine learning libraries, customization options, and diverse deployment choices make it an indispensable tool for professionals across various domains. Streamlit empowers users to effortlessly transform data analyses, machine learning models, and data-driven ideas into interactive web applications. Its user-centric design and active community support have solidified its relevance and importance, making it a go-to solution for those who seek to share insights and make data accessible to a wider audience. Whether you're a data scientist, machine learning engineer, or developer, Streamlit offers a straightforward path to create engaging and impactful web applications, bridging the gap between data and action.
